容器化微服务 Demo 项目开发笔记
小于 1 分钟
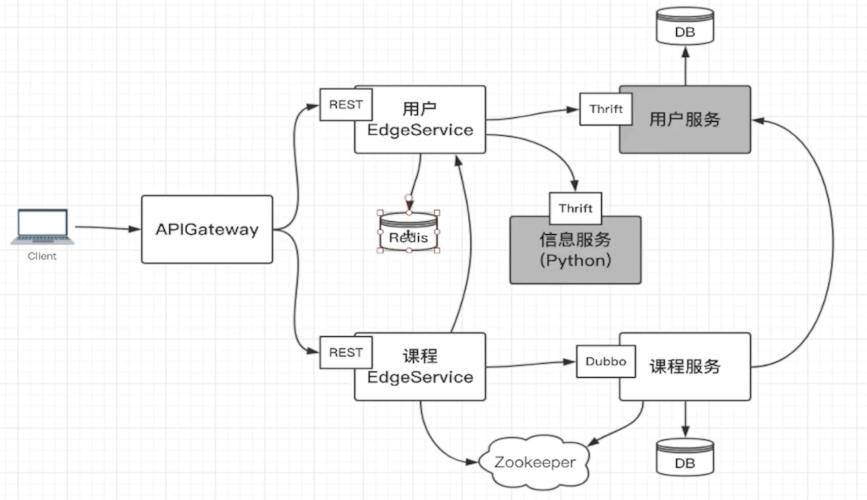
搭建 “课程管理系统” Demo,了解微服务架构玩法。
代码: https://github.com/LawssssCat/blog/tree/master/code/demo-microk8s/
编译 thrift 脚本
#!/bin/bash
SCRIPT_PATH=$(cd $(dirname $0); pwd)
PROJECT_PATH=$(cd $SCRIPT_PATH/../; pwd)
function copy_file() {
local src="$1"
local dst="$2"
mkdir -pv ${dst%/*}
cp -avf $src $dst
}
# message
thrift --gen py -out ${PROJECT_PATH}/message-thrift-python-service/thrift_api/ ${PROJECT_PATH}/script/thrift/message.thrift
thrift --gen java -out ${PROJECT_PATH}/message-thrift-java-api/src/main/java/ ${PROJECT_PATH}/script/thrift/message.thrift
# user
thrift --gen java -out ${PROJECT_PATH}/user-thrift-java-api/src/main/java/ ${PROJECT_PATH}/script/thrift/user.thrift
信息服务(Python)
接口定义语言
namespace java org.example.thrift.message
namespace py message.api
service MessageService {
bool sendMobileMessage(1:string mobile, 2:string message);
bool sendEmailMessage(1:string email, 2:string message);
}
服务器设置
# -*- coding: utf-8 -*-
# thrift 服务器
from thrift_api.message.api import MessageService
from thrift.transport import TSocket, TTransport
from thrift.protocol import TBinaryProtocol
from thrift.server import TServer
class MessageServiceHandler(MessageService.Iface):
def sendMobileMessage(self, mobile, message):
print("sendMobileMessage, mobile:{}, message:{}", mobile, message)
return True
def sendEmailMessage(self, email, message):
# import smtplib
print("sendEmailMessage, email:{}, message:{}", email, message)
return True
if __name__ == '__main__':
# 1
handler = MessageServiceHandler()
processor = MessageService.Processor(handler)
# 2
host = "localhost"
port = 19091
socket = TSocket.TServerSocket(host, port)
# 3
transportFactory = TTransport.TTransportFactoryBase()
# 4
protocolFactory = TBinaryProtocol.TBinaryProtocolFactory()
# server
server = TServer.TSimpleServer(processor, socket, transportFactory, protocolFactory)
print("python thrift server start...")
server.serve()
print("python thrift server exit.")
用户服务
接口实现
接口定义语言
namespace java org.example.thrift.user
struct UserInfo {
1:i32 id,
2:string username,
3:string password,
4:string readName,
5:string mobile,
6:string email,
}
service UserService {
UserInfo getUserById(1:i32 id);
UserInfo getUserByUsername(1:string username);
void registerUser(1:UserInfo userInfo);
}
接口实现
package org.example.thrift.user.service;
import org.apache.thrift.TException;
import org.example.thrift.user.UserInfo;
import org.example.thrift.user.UserService;
import org.example.thrift.user.mapper.UserMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserServiceImpl implements UserService.Iface {
@Autowired
private UserMapper userMapper;
@Override
public UserInfo getUserById(int id) throws TException {
return userMapper.getUserInfoById(id);
}
@Override
public UserInfo getUserByUsername(String username) throws TException {
return userMapper.getUserInfoByUsername(username);
}
@Override
public void registerUser(UserInfo userInfo) throws TException {
userMapper.insertUserInfo(userInfo);
}
}
数据库查询
package org.example.thrift.user.mapper;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
import org.example.thrift.user.UserInfo;
@Mapper
public interface UserMapper {
@Select("select id, username, password, real_name as realName, mobile, email from pe_user where id=#{id}")
UserInfo getUserInfoById(@Param("id")int id);
@Select("select id, username, password, real_name as realName, mobile, email from pe_user where username=#{username}")
UserInfo getUserInfoByUsername(@Param("username")String username);
@Insert("insert into pe_user (username, password, real_name, mobile, email) " +
"values (#{u.username}, #{u.password}, #{u.realName}, #{u.mobile}, #{u.email})")
void insertUserInfo(@Param("u") UserInfo userInfo);
}
数据库定义
DELETE FROM pe_user;
INSERT INTO pe_user (id, username, password, real_name, mobile, email) VALUES
(1, 'james', '!@FASFS@1rsfsa', 'james', '1324121213213', 'test1@gmail.com'),
(2, 'steven1', '!@FAsafd1rsfsa', 'steven', '12312412313', 'steven@gmail.com'),
(3, 'steven2', '$%&^#$FS@1rsfsa', 'steven', '443212313123', 'steven@gmail.com'),
(4, 'steven3', '!@FASfassf#@3fsa', 'steven', '123214123123', 'steven@gmail.com');
数据库数据
DROP TABLE IF EXISTS pe_user;
CREATE TABLE pe_user
(
id integer not null COMMENT '主键ID',
username varchar(255) not null comment '用户名',
password varchar(255) not null COMMENT '用户密码',
real_name varchar(255) NULL DEFAULT null COMMENT '姓名',
mobile varchar(255) NULL DEFAULT NULL COMMENT '手机',
email varchar(255) NULL DEFAULT NULL COMMENT '邮箱',
primary key(id)
);
服务器
package org.example.thrift.user.thrift;
import lombok.extern.slf4j.Slf4j;
import org.apache.thrift.protocol.TBinaryProtocol;
import org.apache.thrift.server.TServer;
import org.apache.thrift.server.TSimpleServer;
import org.apache.thrift.transport.TServerSocket;
import org.apache.thrift.transport.TTransportException;
import org.apache.thrift.transport.TTransportFactory;
import org.example.thrift.user.UserService;
import org.example.thrift.user.service.UserServiceImpl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
@Slf4j
@Configuration
public class ThriftServer {
@Value("${thrift.server.port}")
private int thriftServerPort;
@Autowired
private UserServiceImpl userServiceImpl;
public void startThriftServer() {
UserService.Processor<UserServiceImpl> userServiceProcessor = new UserService.Processor<>(userServiceImpl);
try (TServerSocket socket = new TServerSocket(thriftServerPort)){
TServer.Args serverArgs = new TServer.Args(socket);
serverArgs.processor(userServiceProcessor);
serverArgs.protocolFactory(new TBinaryProtocol.Factory());
serverArgs.transportFactory(new TTransportFactory());
// server
TSimpleServer server = new TSimpleServer(serverArgs);
log.info("TServer - Start serving...");
server.serve();
} catch (TTransportException e) {
throw new RuntimeException(e);
}
}
}